CSC204 - Project 2
Swarming with Objects
Assigned April 8th
Due April 15th 4:45pm
Overview
In this project, you will implement a Java program to display swarming patterns and
properties of social networks.
Swarms
Some of the simplest objects we can explore involve simulating the behavior of animals. Our choice
for this project will be Ants. Scientists study the simple behavior of ants, termites, fish, and
other animals to try and learn rules for crowds and social interaction.
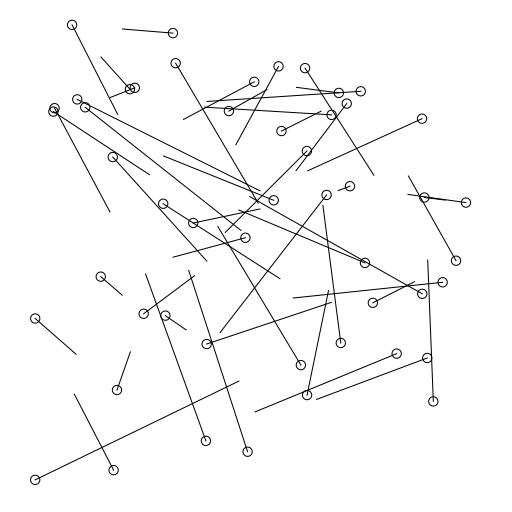
Two swarms of ants following the leader
An ant is defined to be at a particular point in space, specified by x and y coordinates. Each
ant is also given one friend, someone else (not themselves) to follow around. Every second, an ant
will perform three behaviors
- Move 1/2 the distance between itself and its friend.
- Wiggle and move randomly.
- Move away from the closest Ant if that Ant is too close.
Your task is to create two instantiable classes which capture an x,y Point, and an Ant which will
swarm. Through these objects, you will be able to explore the grouping effects of social networks.
In particular, you can study how many groups are formed through these simple rules of follow the
leader.
What to do
You have been given the main method of Swarm.java. The comments in this
class explain the steps of reading data from the user, initializing all of the Ants, and running
the simulation as described above.
Point Class
The first class you will implement is Point.java
Data Members
double x
stores the x coordinate
double y
stores the y coordinate
Methods
public Point(double x, double y)
is the constructor, and sets the x and y
coordinates.
public double getX()
returns the object's x value.
public double getY()
returns the object's y value.
public void setX(double x)
sets the x value to be the input x.
public void setY(double y)
sets the y value to be the input y.
public double distance(Point p)
Calculates the Euclidean distance between two
points, using the formula sqrt((x1 - x2) ^ 2 + (y1 - y2) ^ 2).
You can add a main method to this class for unit testing, and should, but it is not necessary.
Ant Class
The second class you will implement is Ant.java
Data Members
Point pos
stores the current position of the Ant
Ant friend
stores the current friend of the Ant
Methods
public Ant(double x, double y)
is the constructor, and initialized the Point pos to
be at x, y.
public void addFriend(Ant f)
sets the value of friend to be the input f.
public double getX()
returns the Ant's x value.
public double getY()
returns the Ant's y value.
public Point getPos()
returns the Ant's Position.
public void draw()
draws the Ant to the StdDraw screen. I used a circle centered at
the Point of pos, and with a radius of 0.05. Also draws the path the Ant will follow, between it's
current location and 1/2 the distance to it's friend.
public void move()
moves the Ant 1/2 the distance toward it's friend.
public void wiggle(double w)
randomly changes the x and y position. This should add or
subtract a random number from each by w/2.
public void repel(Ant other)
moves the Ant away from the other Ant by twice their
current distance. This should be in the direction exactly opposite of the other Ant.
You can add a main method to this class for unit testing, and should, but it is not necessary.
How to approach this project
I recommend working from the bottom up. First, create the Point class and test all of its methods.
Then create an Ant class, which uses Points, and test all of its methods. Use stubs to make code
that will compile but have limited functionality. This will let you work on the move method
separate from the wiggle or repel methods.
What to turn in
You should hand in three files to your file space on the cs.centenary.edu server, following the
instructions of Lab 1. The files are
- Swarm.java
- Ant.java
- Point.java
Place these files in a directory called project2
.